import random
hands = ['グー', 'チョキ', 'パー']
view = [0,1,2,3,4,5,6,7,8]
results = {'win':'プレイヤー1が勝ち', 'lose':'プレイヤー2が勝ち', 'draw':'あいこ'}
player = {'player1':'プレイヤー1', 'player2':'プレイヤー2'}
def start_message():
print('〇×ゲームスタート!!')
print('最初にどちらがプレイヤー1、プレイヤー2にするか決めてください')
print('先攻後攻をじゃんけんで決めてください')
# 先攻後攻決め
def get_my_hand():
return int(input('0:グー, 1:チョキ, 2:パー'))
def get_you_hand():
return random.randint(0, 2)
def get_hand_name(hand_number):
return hands[hand_number]
def view_hand(my_hand, you_hand):
print('プレイヤー1の手は' + get_hand_name(my_hand))
print('プレイヤー2の手は' + get_hand_name(you_hand))
def get_result(hand_diff):
if hand_diff == 0:
print('もう一回してください')
return 'draw'
elif hand_diff == -1 or hand_diff == 2:
print('プレイヤー1が先攻(〇)')
print('プレイヤー2が後攻(×)')
return 'win'
else:
print('プレイヤー1が後攻(×)')
print('プレイヤー2が先攻(〇)')
return 'lose'
def view_result(result):
print(results[result])
# 表を表示
def view_table():
print('ーーーーー')
for i in range(0, len(view)):
if(i%3 == 2):
print(view[i])
print('ーーーーー')
elif(i%3 == 1):
print(' | ' + str(view[i]) + ' | ', end='')
else :
print(view[i], end='')
print()
# プレイヤー1が先攻の場合
def game(choice):
if(choice == '〇'):
message = int(input("先攻はプレイヤー1です。どこに〇を配置しますか?(例:0) "))
elif(choice == '×'):
message = int(input("後攻はプレイヤー2です。どこに×を配置しますか?(例:0) "))
if(view[message] == '〇' or view[message] == '×'):
game(choice)
else:
view[message] = choice
# プレイヤー2が先攻の場合
def game2(choice):
if(choice == '〇'):
message = int(input("先攻はプレイヤー2です。どこに〇を配置しますか?(例:0) "))
elif(choice == '×'):
message = int(input("後攻はプレイヤー1です。どこに×を配置しますか?(例:0) "))
if(view[message] == '〇' or view[message] == '×'):
game(choice)
else:
view[message] = choice
def judgement():
#縦の勝ち手
if view[0] == view[1] == view[2]:
return view[0]
if view[3] == view[4] == view[5]:
return view[3]
if view[6] == view[7] == view[8]:
return view[6]
#横の勝ち手
if view[0] == view[3] == view[6]:
return view[0]
if view[1] == view[4] == view[7]:
return view[1]
if view[2] == view[5] == view[8]:
return view[2]
#斜めの勝ち手
if view[0] == view[4] == view[8]:
return view[0]
if view[2] == view[4] == view[6]:
return view[2]
else:
return None
def play():
# じゃんけん先攻後攻決め
my_hand = get_my_hand()
you_hand = get_you_hand()
hand_diff = my_hand - you_hand
view_hand(my_hand, you_hand)
result = get_result(hand_diff)
view_result(result)
# あいこの場合
if result == 'draw':
play()
# これより下が〇×ゲーム実行
if result == 'win':
view_table()
for i in range(0,9):
if(i %2 == 0) : #プレイヤー1先攻
print('プレイヤー1')
game('〇')
else: #プレイヤー2後攻
print('プレイヤー2')
game("×")
view_table()
if judgement(): #勝敗を判定
print(judgement() + 'の勝ち!')
break
if i == 8: #引き分け
print('引き分け')
break
if result == 'lose':
view_table()
for i in range(0,9):
if(i %2 == 0) : #プレイヤー2先攻
print('プレイヤー2')
game2('〇')
else: #プレイヤー1後攻
print('プレイヤー1')
game2("×")
view_table()
if judgement(): #勝敗を判定
print(judgement() + 'の勝ち!')
break
if i == 8: #引き分け
print('引き分け')
break
start_message()
play()
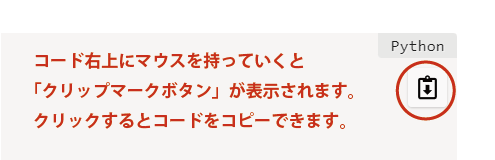