import datetime
import json
# ユーザーデータの読み込み
def load_users():
try:
with open('users.json', 'r') as f:
return json.load(f)
except FileNotFoundError:
return {}
# ユーザーデータの保存
def save_users(users):
with open('users.json', 'w') as f:
json.dump(users, f)
# ユーザー認証
def authenticate(users):
user_id = input("ユーザーIDを入力してください: ")
password = input("パスワードを入力してください: ")
if user_id in users and users[user_id]['password'] == password:
print(f"ようこそ、{user_id}さん")
return user_id
else:
print("認証に失敗しました。")
return None
# 打刻機能
def punch_clock(users, user_id, punch_type):
now = datetime.datetime.now()
if user_id not in users:
users[user_id] = {'password': '', 'punches': []}
users[user_id]['punches'].append({'type': punch_type, 'time': now.isoformat()})
print(f"{punch_type}打刻: {now}")
save_users(users)
# 勤務時間の表示
def display_times(users, user_id):
if user_id not in users or not users[user_id]['punches']:
print("打刻データがありません。")
return
for punch in users[user_id]['punches']:
print(f"{punch['type']} - {punch['time']}")
# メイン処理
users = load_users()
user_id = authenticate(users)
if user_id:
while True:
print("\n1. 出勤打刻\n2. 退勤打刻\n3. 休憩開始打刻\n4. 休憩終了打刻\n5. 勤務時間表示\n6. 終了")
choice = input("選択肢を入力してください: ")
if choice == '1':
punch_clock(users, user_id, '出勤')
elif choice == '2':
punch_clock(users, user_id, '退勤')
elif choice == '3':
punch_clock(users, user_id, '休憩開始')
elif choice == '4':
punch_clock(users, user_id, '休憩終了')
elif choice == '5':
display_times(users, user_id)
elif choice == '6':
break
else:
print("無効な選択です。")
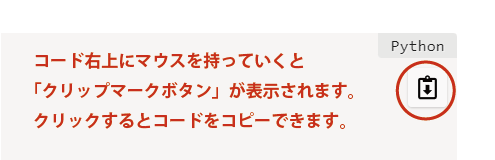